@rabarar you need to modify to fit your needs. I haven’t looked at this code in a while. Check out my original post where I discussed it.
Here’s the summary:
Sending SMS
One of the cooler and more familiar features of the nRF9160 Feather is the ability for it to send SMS messages! Sending an SMS message is as simple as invoking a few AT commands to the nRF9160 Feather modem firmware. The only drawback is that your nRF9160 Feather must have the v1.1.2 or newer modem firmware installed (A.K.A MFW v1.2.2). Theres a full section in the nRF9160 Feather documentation about how to update the modem firmware.
A great way to start with SMS is the provided example within the nrf9160-feather/samples/sms/
directory. Let’s dissect what’s inside:
One of the most important things you’ll see is at_commands
. These commands are the commands you’ll get familiar with in order to send a message. They look like gibberish, but I promise you they’re important!
const char *at_commands[] = {
"AT+CNMI=3,2,0,1",
"AT+CMGS=21\r0001000B912131445411F0000009C834888E2ECBCB21\x1A",
/* Add more here if needed */
};
The first line readies the nRF9160 Feather to send an SMS. While the second is the message itself. Text messages use a special encoding which don’t make them terribly easy for a human to read. In the case above, the above message will look something like this to the receiver:
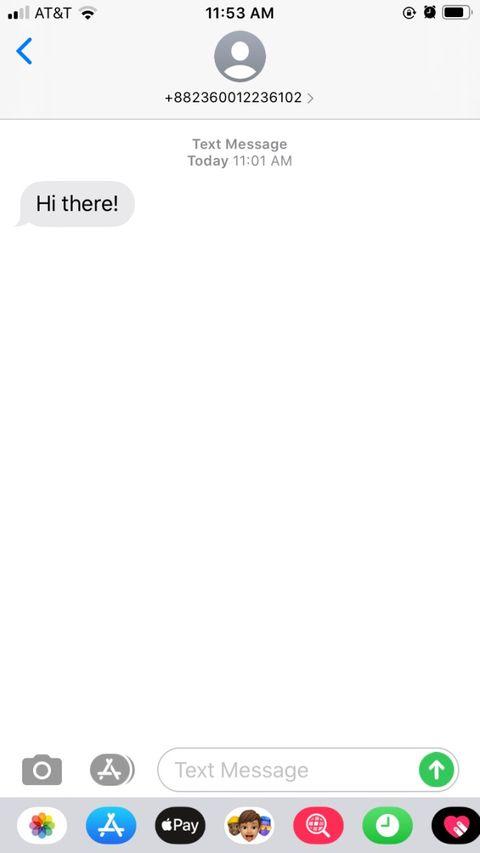
But how do we control or generate this special format? Currently there is no built-in PDU encoder in Zephyr/nRF Connect SDK. You can use an external one like this one to generate your messages though!
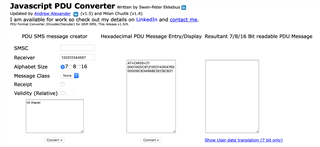
To get the correct format, update the Receiver text box with the target phone number. Then update your message and hit Convert. It will spit out the encoded message. This command relies heavily on special characters, you need to make sure that they do not get removed. In particular, you need to have a carriage return (\r
) after AT+CMGS=21
and \x1A
must be always at the end of the message. Again, check out the at_commands
array above to see exactly how it looks like.
The rest of the sms
is very similar to the button and deep_sleep
examples. The idea being is that when you press the button, you’ll invoke the AT commands using a work function. Here’s the work function that gets called when you press the Mode button:
/* Work for starting sms commands*/
static void sms_work_fn(struct k_work *work)
{
for (int i = 0; i < ARRAY_SIZE(at_commands); i++)
{
int err;
enum at_cmd_state cmd_state = AT_CMD_OK;
printk("%s\r\n", at_commands[i]);
if ((err = at_cmd_write_with_callback(at_commands[i],
at_cmd_handler,
&cmd_state)) != 0)
{
printk("Error sending: %s. Error: %i. State: %i.", at_commands[i], err, (int)cmd_state);
}
}
/* Run SMS work */
/* k_delayed_work_submit(&sms_work, K_SECONDS(300)); */
}
The sms_work_fn
iterates through all the commands and sends them to the modem.